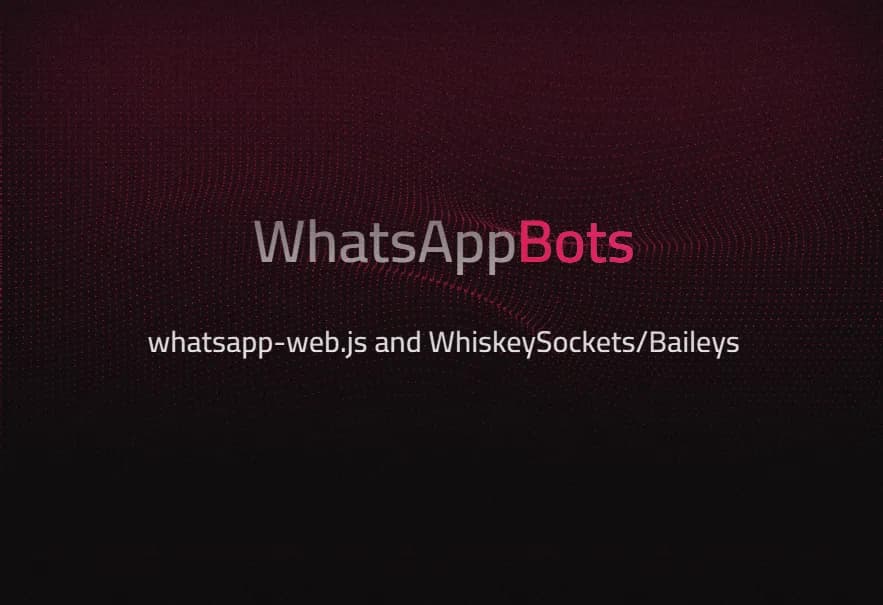
WhatsApp bots have become increasingly popular for automating tasks, customer service, and managing group interactions. In this blog post, we'll compare two popular libraries for creating WhatsApp bots: whatsapp-web.js
and WhiskeySockets/Baileys
. We'll also build a simple bot using TypeScript and Bun.
Feature | whatsapp-web.js | Baileys |
---|---|---|
Protocol Used | WhatsApp Web (Puppeteer) | WhatsApp WebSocket (Reverse-engineered) |
Requires Chrome? | ✅ Yes | ❌ No |
Scalability | ❌ Limited | ✅ Highly scalable |
Multi-session support | ❌ No | ✅ Yes |
Performance | ❌ Higher resource consumption | ✅ Lightweight and efficient |
Stability | ✅ More stable (official Web implementation) | ❌ More prone to breaking due to API changes |
Attachment Handling | ✅ Requires manual handling (Users must manually download, encode, and format media before sending. Additionally, sending videos and GIFs may require using a separately installed Chrome browser. More info.) | ✅ Supports attachments natively |
Ease of Setup | ✅ Easier to set up | ❌ Slightly more complex |
Both libraries have their strengths: whatsapp-web.js
is easier to get started with, while Baileys
is more scalable and lightweight. However, Baileys
users may need to update their implementation more frequently due to WhatsApp API changes.
For this example, we'll create a simple bot using both whatsapp-web.js
and Baileys
.
sh
bun add whatsapp-web.js qrcode-terminal baileys
typescript
//whatsapp-web.ts
import { Client, LocalAuth, MessageMedia } from "whatsapp-web.js";
import qrcode from "qrcode-terminal";
import fs from "fs";
const client = new Client({
authStrategy: new LocalAuth(),
});
client.on("qr", (qr) => {
console.log("Scan the QR code to log in:");
qrcode.generate(qr, { small: true });
});
client.on("ready", () => {
console.log("Bot is ready!");
});
client.on("message", async (msg) => {
if (msg.body.toLowerCase() === "hello") {
await msg.reply("Hello! How can I help you?");
}
if (msg.body.toLowerCase() === "send image") {
const media = MessageMedia.fromFilePath("./image.jpg");
await client.sendMessage(msg.from, media, { caption: "Here is your image!" });
}
});
client.initialize();
typescript
//baileys.ts
import makeWASocket, { useMultiFileAuthState, MessageType, WAMediaUpload } from "@whiskeysockets/baileys";
import fs from "fs";
async function startBaileysBot() {
const { state, saveCreds } = await useMultiFileAuthState("auth_info");
const sock = makeWASocket({
auth: state,
});
sock.ev.on("creds.update", saveCreds);
sock.ev.on("connection.update", (update) => {
const { connection, lastDisconnect } = update;
if (connection === "close") {
console.log("Connection closed, reconnecting...");
startBaileysBot();
} else if (connection === "open") {
console.log("Bot is ready!");
}
});
sock.ev.on("messages.upsert", async (m) => {
const message = m.messages[0];
if (!message.key.fromMe && message.message?.conversation === "hello") {
await sock.sendMessage(message.key.remoteJid!, { text: "Hello! How can I help you?" });
}
if (!message.key.fromMe && message.message?.conversation === "send image") {
const buffer = fs.readFileSync("./image.jpg");
await sock.sendMessage(message.key.remoteJid!, { image: buffer, caption: "Here is your image!" });
}
});
}
startBaileysBot();
For whatsapp-web.js
:
sh
bun run whatsapp-web.ts
For Baileys
:
sh
bun run baileys.ts
If you're building a small bot, whatsapp-web.js
is a great choice. However, for more advanced use cases, Baileys
is a better option due to its efficiency and multi-device support. That said, Baileys
users should be prepared for potential API-breaking changes. Now you have examples of both, including attachment handling, so you can choose the one that fits your needs best!